Maven Guide
Maven Guide
https://www.baeldung.com/maven-goals-phases https://stackoverflow.com/questions/16205778/what-are-maven-goals-and-phases-and-what-is-their-difference
https://www.baeldung.com/maven
lifecycle
- default: the main life cycle as it's responsible for project deployment
- clean: to clean the project and remove all files generated by the previous build
- site: to create the project's site documentation
Each life cycle consists of a sequence of phases
Phase
A Maven phase represents a stage in the Maven build lifecycle.
- validate: check if all information necessary for the build is available
- compile: compile the source code
- test-compile: compile the test source code
- test: run unit tests
- package: package compiled source code into the distributable format (jar, war, …)
- integration-test: process and deploy the package if needed to run integration tests
- install: install the package to a local repository
- deploy: copy the package to the remote repository
mvn
This won't only execute the specified phase but all the preceding phases as well.
Goal
Each phase is a sequence of goals, and each goal is responsible for a specific task.
Here are some of the phases and default goals bound to them:
- compiler:compile – the compile goal from the compiler plugin is bound to the compile phase
- compiler:testCompile is bound to the test-compile phase
- surefire:test is bound to test phase
- install:install is bound to install phase
- jar:jar and war:war is bound to package phase
Plugin
A Maven plugin is a group of goals. However, these goals aren't necessarily all bound to the same phase.
mvn install
This will execute the entire default lifecycle. Alternatively, we can stop at the install phase:
mvn clean install
To clean the project first – by running the clean lifecycle – before the new build.
mvn compiler:compile
We can also run only a specific goal of the plugin:
Maven archetype
mvn archetype:generate -DgroupId=com.seashell -DartifactId=my-webapp -DarchetypeArtifactId=maven-archetype-webapp
mvn archetype:generate -DgroupId=com.seashell -DartifactId=my-webapp -DarchetypeArtifactId=maven-archetype-webapp |
Directory Layout
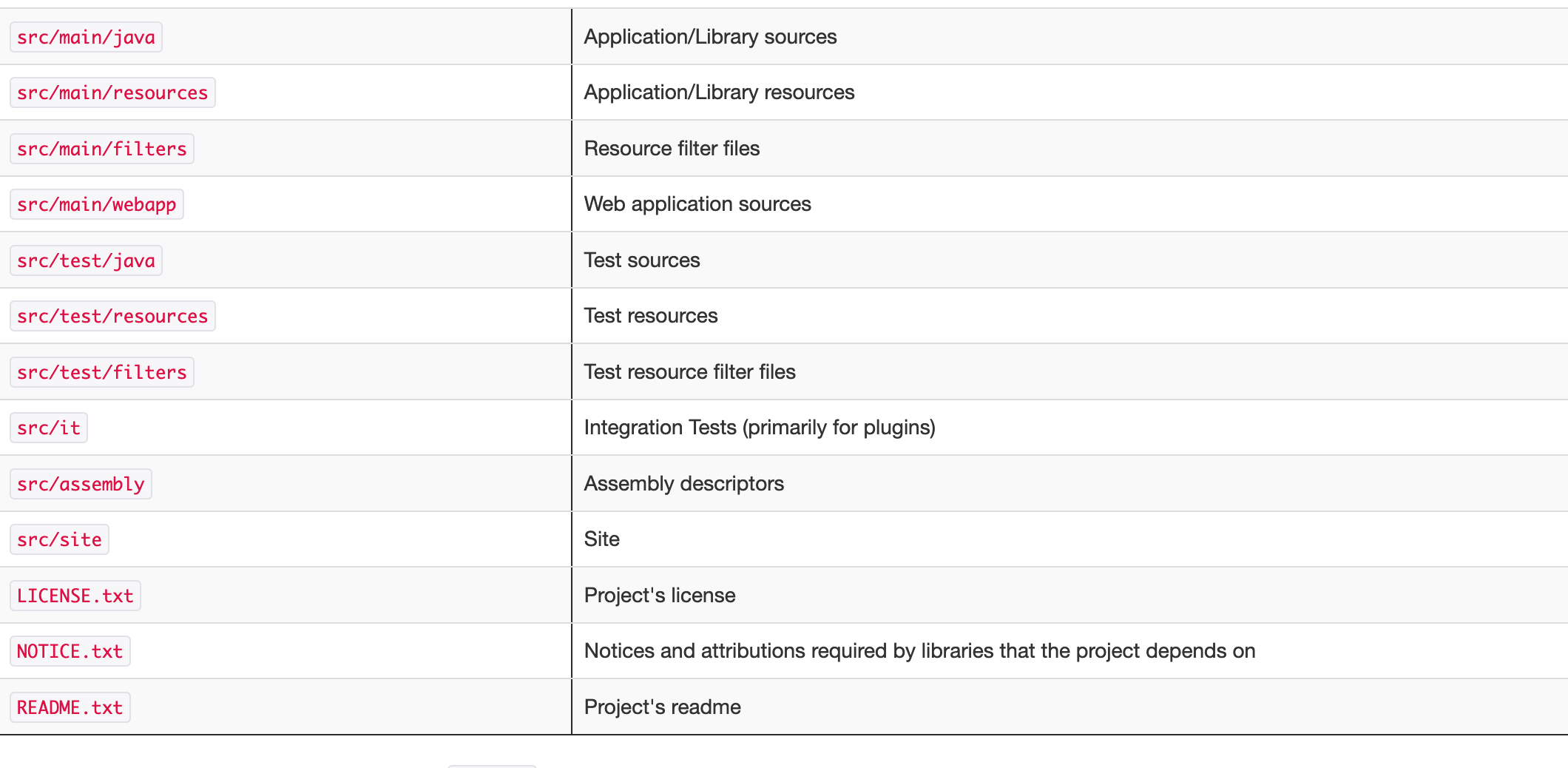
denpendency
https://maven.apache.org/plugins/maven-dependency-plugin/tree-mojo.html
mvn dependency:tree
Plugin Configuration
compliler plugin
https://www.baeldung.com/maven-java-version
https://www.baeldung.com/maven-compiler-plugin
https://mkyong.com/maven/how-to-tell-maven-to-use-java-8/
war plugin
https://maven.apache.org/plugins/maven-war-plugin/
https://maven.apache.org/plugins/maven-war-plugin/usage.html
- using the
package
phase with the project package type aswar
- invocation of the
war:war
goal
Project Structure
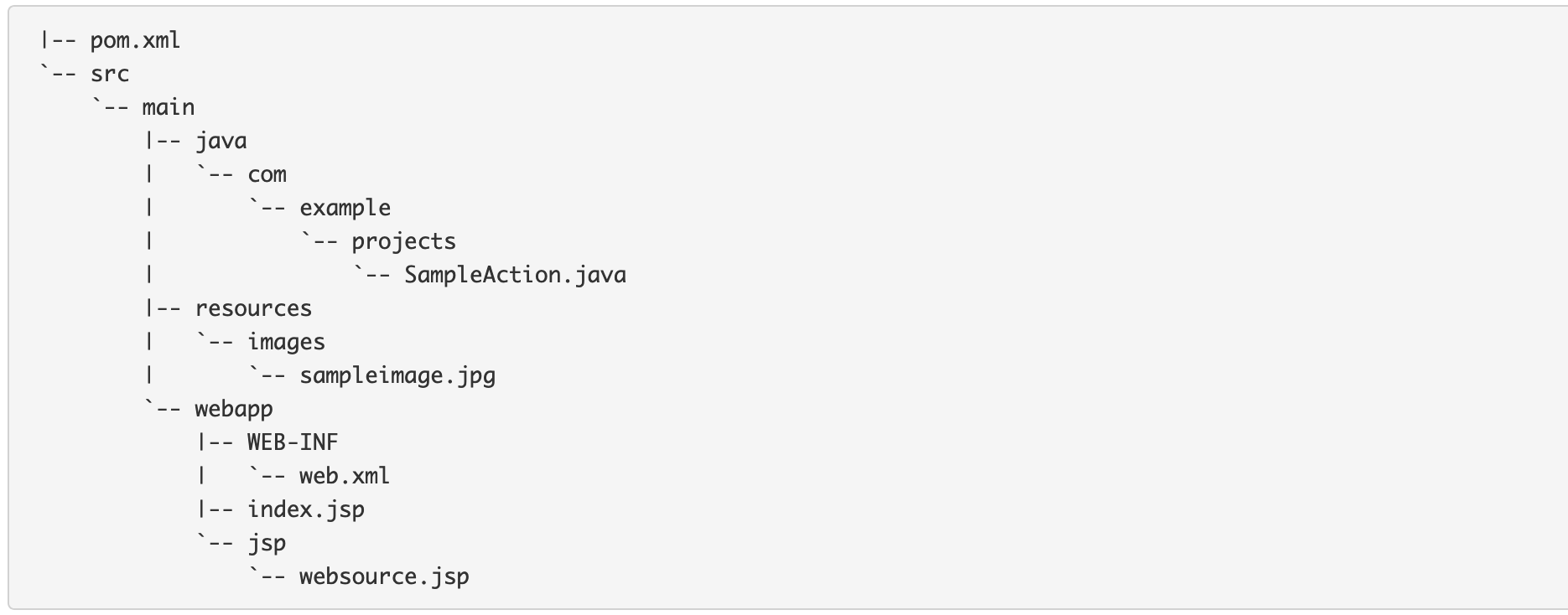
mvn package |
Output: target/xxx.war
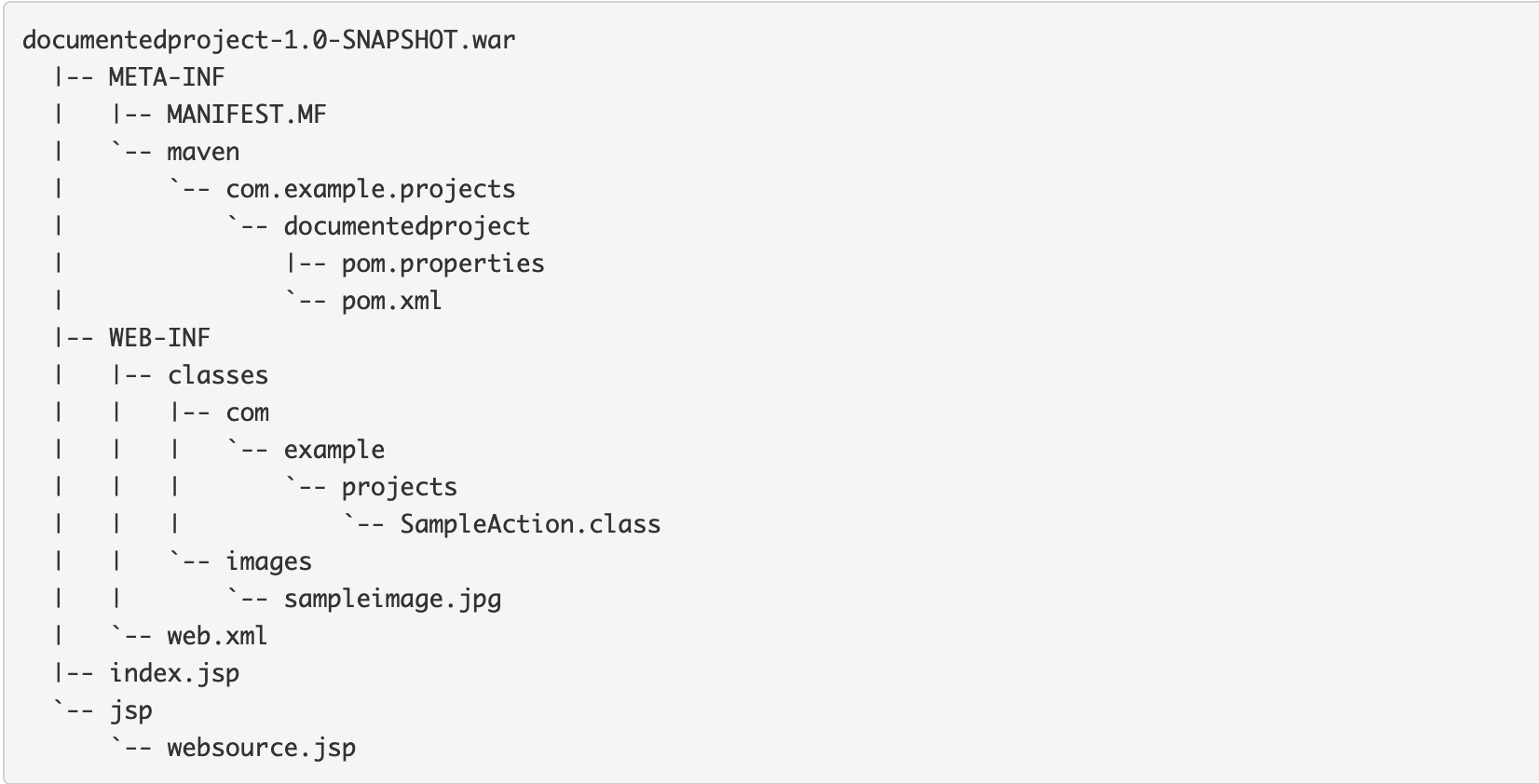