RabbitMQ之tutorial-2
RabbitMQ之tutorial-2
Tutorial Two
https://www.rabbitmq.com/tutorials/tutorial-two-python.html
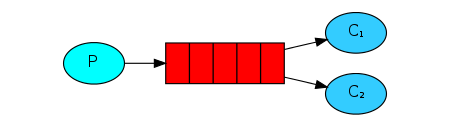
The main idea behind Work Queues (aka: Task Queues) is to avoid doing a resource-intensive task immediately and having to wait for it to complete
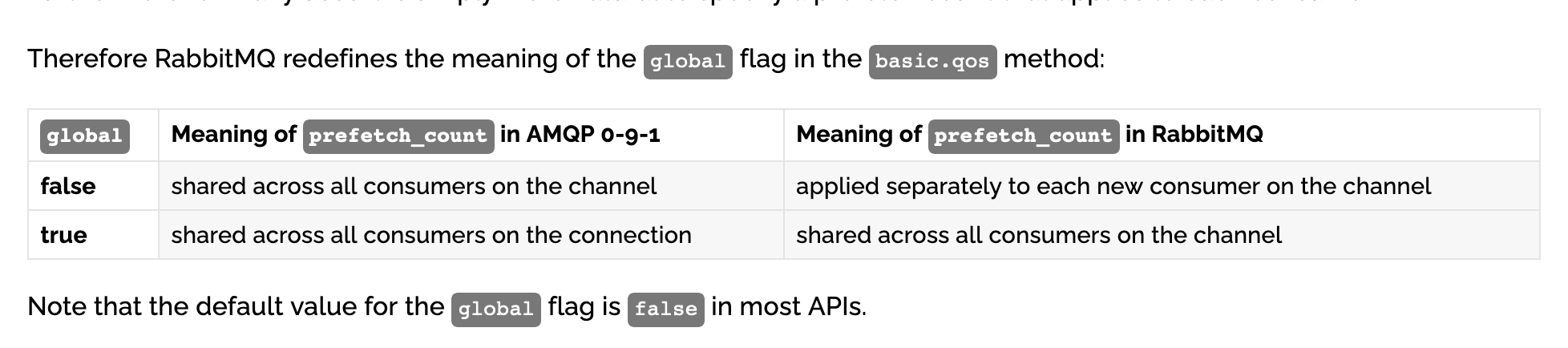
new_task.py
import pika |
worker.py
import pika |
Round-robin dispatching
By default, RabbitMQ will send each message to the next consumer, in sequence. On average every consumer will get the same number of messages.
Terminal1 python worker.py
Terminal2 python worker.py
Terminal3 python new_task.py First message. python new_task.py Second message.. python new_task.py Third message... python new_task.py Fourth message.... python new_task.py Fifth message.....
Message acknowledgment
we don't want to lose any tasks. If a worker dies, we'd like the task to be delivered to another worker.
There aren't any message timeouts; RabbitMQ will redeliver the message when the consumer dies. It's fine even if processing a message takes a very, very long time.
Acknowledgement must be sent on the same channel that received the delivery.
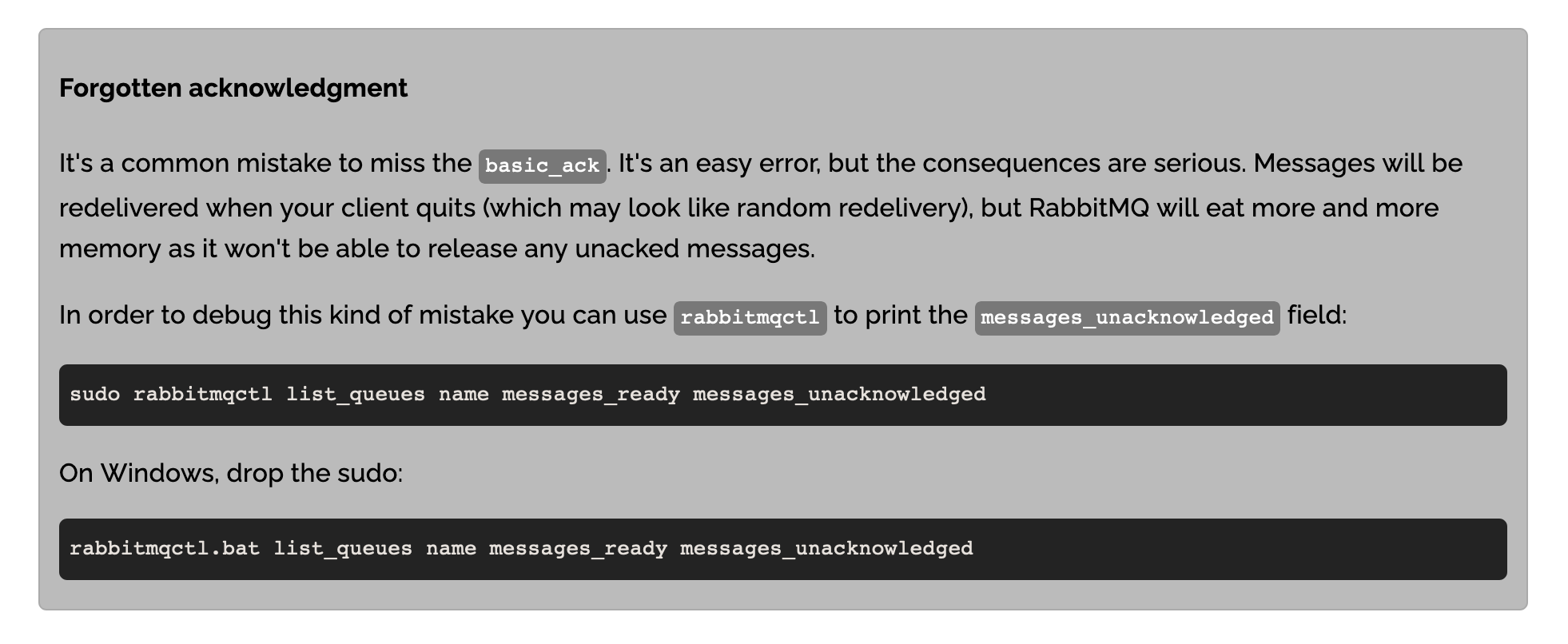
Message durability
We have learned how to make sure that even if the consumer dies, the task isn't lost. But our tasks will still be lost if RabbitMQ server stops.
When RabbitMQ quits or crashes it will forget the queues and messages unless you tell it not to. Two things are required to make sure that messages aren't lost: we need to mark both the queue and messages as durable.
Fair Dispatch
It just blindly dispatches every n-th message to the n-th consumer.
use the Channel#basic_qos
channel method with the prefetch_count=1 setting,tell RabbitMQ not to give more than one message to a worker at a time. Or, in other words, don't dispatch a new message to a worker until it has processed and acknowledged the previous one. Instead, it will dispatch it to the next worker that is not still busy.
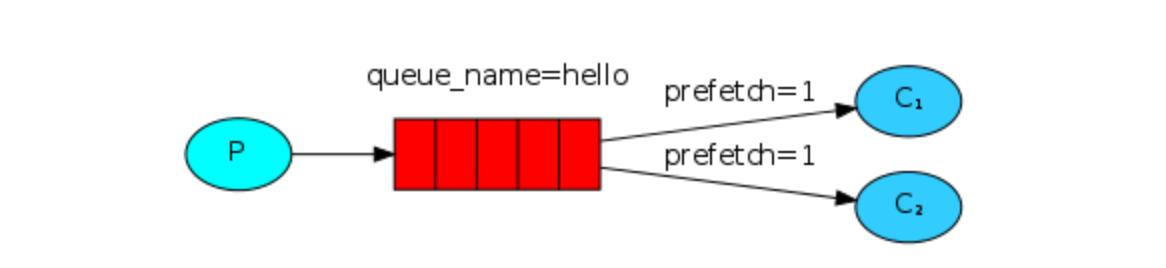
If all workers are busy, queue can fill up.
- add more worker
- message TTL